When developing applications with React, ensuring accessibility for all users, including those relying on screen readers, is essential. One key aspect of this is understanding how JSX (JavaScript XML) can affect the user experience of a screen reader due to how JSX renders in the DOM (Document Object Model).
Understanding the Issue
Consider the following JSX code snippet:
<h1> This is a sentence about {hello}, {world}! </h1>
Which renders in the DOM in fragments, like this:
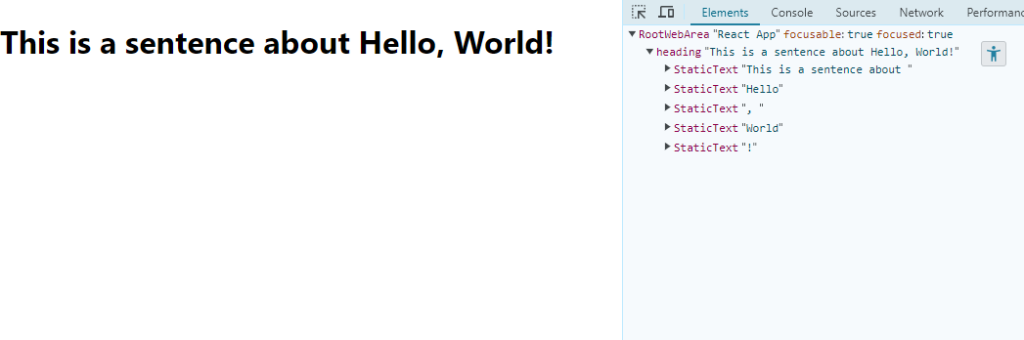
For users navigating with Narrator on Windows, this breaks up the header into multiple navigable parts. Instead of being able to navigate the entire sentence in one go, the screen reader forces the user to navigate through each fragmented section. This behavior disrupts the natural flow of reading and might be unexpected when switching between sites that don’t rely on JSX.
On a Mac, using VoiceOver (VO), this becomes even more problematic. VO will read this as:
“Heading level 1, this is a sentence about, level 2, hello, level 2, , , level 2, world, level 2, !”
This verbosity and fragmentation make it hard for users to comprehend the content.
The Solution: Using Template Literals
To address this issue, we can use template literals to force the DOM to read the sentence as a single string. This approach allows for dynamic text while maintaining the expected behavior for screen readers.
<h1>{`This is a sentence about ${hello}, ${world}!`}</h1>
Now, the entire sentence is treated as a single string. As a result, screen readers will read this as a continuous piece of text, improving the overall experience.
Benefits of Using Template Literals
- Improved Readability: Screen readers will read the entire sentence as intended, providing a smoother and more understandable experience.
- Consistency Across Devices: Both Windows and Mac users will benefit from a consistent and logical reading order.
- Dynamic Content: Template literals allow you to insert dynamic text without compromising accessibility.
Conclusion
Ensuring a seamless experience for screen reader users when working with React and JSX is crucial. By using template literals to render complete sentences, developers can significantly enhance accessibility and usability. This small adjustment can make a big difference in how users interact with your application, leading to a more inclusive and enjoyable experience for all.
Implement these best practices in your React projects to support a wider audience and uphold the principles of accessible web development.